“==” Checking
When we create any object there are two parts to the object one is the content and the other is reference to that content.
So for example if you create an object as shown in below code:-
-
“.NET interview questions” is the content.
-
“o” is the reference to that content.


When you are using string data type it always does content comparison. In other words you either use “.Equals()” or “==” it always do content comparison.
Note:
- Always use “Equal” or “Reference Equal” methods when compare to Reference type.
- Use “==” operator when use Value types.
- Equal method will compare Contents when we use in Value Types comparison, and will compare Reference when we use Reference Types.
- “==” will do reference comparison when we compare two “Objects” type which points “String” type or some other types.
- “Equal” method will do value comparison when we compare two “Objects” type which points “String” content.
Object.Equals
Determines whether the specified object is equal to the current object. The type of comparison between the current instance and the obj parameter depends on whether the current instance is a reference type or a value type. If the current instance is a reference type, the Equals(Object) method tests for reference equality, and a call to the Equals(Object) method is equivalent to a call to the ReferenceEquals method.
Reference equality means that the object variables that are compared refer to the same object. The following example illustrates the result of such a comparison. It defines a Person class, which is a reference type, and calls the Person class constructor to instantiate two new Person objects, person1a and person2, which have the same value. It also assigns person1a to another object variable, person1b. As the output from the example shows, person1a and person1b are equal because they reference the same object. However, person1a and person2 are not equal, although they have the same value

If the current instance is a value type, the Equals(Object) method tests for value equality. Value equality means the following. The two objects are of the same type. As the following example shows, a Byte object that has a value of 12 does not equal an Int32 object that has a value of 12, because the two objects have different run-time types.

The values of the public and private fields of the two objects are equal. The following example tests for value equality. It defines a Person structure, which is a value type, and calls the Person class constructor to instantiate two new Person objects, person1 and person2, which have the same value. As the output from the example shows, although the two object variables refer to different objects, person1 and person2 are equal because they have the same value for the private personName field.
Notes for Callers
Derived classes frequently override the Object.Equals(Object) method to implement value equality. In addition, types also frequently provide an additional strongly typed overload to the Equals method, typically by implementing the IEquatable<T> interface. When you call the Equals method to test for equality, you should know whether the current instance overrides Object.Equals and understand how a particular call to an Equals method is resolved. Otherwise, you may be performing a test for equality that is different from what you intended, and the method may return an unexpected value.
The following example provides an illustration. It instantiates three StringBuilder objects with identical strings, and then makes four calls to Equals methods. The first method call returns true, and the remaining three return false.
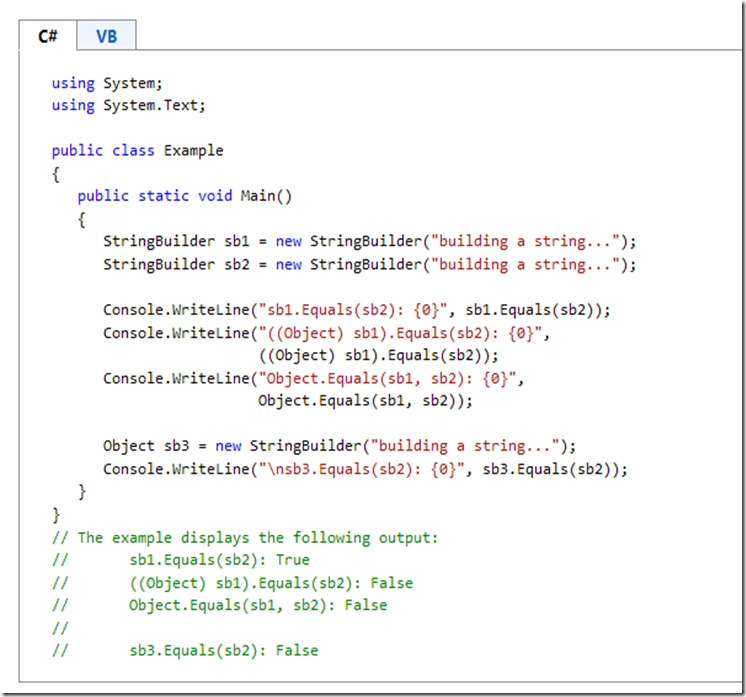
Object.ReferenceEquals
Determines whether the specified Object instances are the same instance.
Unlike the Equals method and the equality operator, the ReferenceEquals method cannot be overridden. Because of this, if you want to test two object references for equality and you are unsure about the implementation of the Equals method, you can call the ReferenceEquals method.
However, the return value of the ReferenceEquals method may appear to be anomalous in these two scenarios:
When comparing value types. If objA and objB are value types, they are boxed before they are passed to the ReferenceEquals method. This means that if both objA and objB represent the same instance of a value type, the ReferenceEquals method nevertheless returns false, as the following example shows.
When comparing strings. If objA and objB are strings, the ReferenceEquals method returns true if the string is interned. It does not perform a test for value equality. In the following example, s1 and s2 are equal because they are two instances of a single interned string. However, s3 and s4 are not equal, because although they are have identical string values, that string is not interned.

String.IsInterned Method
Retrieves a reference to a specified String. The common language runtime automatically maintains a table, called the intern pool, which contains a single instance of each unique literal string constant declared in a program, as well as any unique instance of String you add programmatically by calling the Intern method.
The intern pool conserves string storage. If you assign a literal string constant to several variables, each variable is set to reference the same constant in the intern pool instead of referencing several different instances of String that have identical values.
This method looks up str in the intern pool. If str has already been interned, a reference to that instance is returned; otherwise, null is returned. Compare this method to the Intern method. This method does not return a Boolean value. If you call the method because you want a Boolean value that indicates whether a particular string is interned, you can use code such as the following.

IEquatable<T> Interface
Defines a generalized method that a value type or class implements to create a type-specific method for determining equality of instances. The implementation of the Equals method is intended to perform a test for equality with another object of type T, the same type as the current object. The Equals method is called in the following circumstances:
· When the Equals method is called and the other parameter represents a strongly-typed object of type T. (If other is of type Object, the base Object.Equals(Object) method is called. Of the two methods, IEquatable<T>.Equals offers slightly better performance.)
· When the search methods of a number of generic collection objects are called. Some of these types and their methods include the following:
· Some of the generic overloads of the BinarySearch method.
· The search methods of the List<T> class, including List<T>.Contains(T), List<T>.IndexOf, List<T>.LastIndexOf, and List<T>.Remove.
· The search methods of the Dictionary<TKey, TValue> class, including ContainsKey and Remove.
· The search methods of the generic LinkedList<T> class, including LinkedList<T>.Contains and Remove.
In other words, to handle the possibility that objects of a class will be stored in an array or a generic collection object, it is a good idea to implement IEquatable<T> so that the object can be easily identified and manipulated. When implementing the Equals method, define equality appropriately for the type specified by the generic type argument. For example, if the type argument is Int32, define equality appropriately for the comparison of two 32-bit signed integers.



Note:
Below .NET internal classes and objects would be created dynamically during the call of EQUALITY checking on objects at runtime.




List Object’s Contains method which will call these above objects and classes during runtime. There are lot of Search and Compare methods eventually will use these .NET runtime objects.

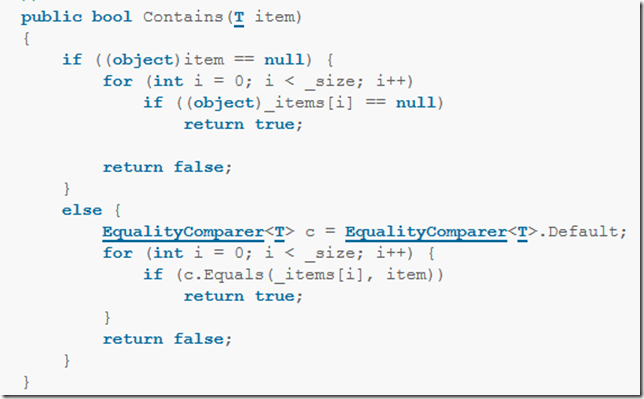
IComparable<T> Interface
Defines a generalized comparison method that a value type or class implements to create a type-specific comparison method for ordering instances.
The IComparable<T> interface defines the CompareTo method, which determines the sort order of instances of the implementing type. The IEquatable<T> interface defines the Equals method, which determines the equality of instances of the implementing type.
The following code example illustrates the implementation of IComparable<T> for a simple Temperature object. The example creates a SortedList<TKey, TValue> collection of strings with Temperature object keys, and adds several pairs of temperatures and strings to the list out of sequence. In the call to the Add method, the SortedList<TKey, TValue> collection uses the IComparable<T> implementation to sort the list entries, which are then displayed in order of increasing temperature.


Note:
Below .NET internal classes and objects would be created dynamically during the call of COMPARE (or) Sorting operation on objects at runtime.



Blogger Labels:
Object,
Comparable,
Equals,
Determines,
comparison,
instance,
parameter,
reference,
method,
ReferenceEquals,
example,
Person,
output,
Value,
Byte,
Notes,
Callers,
addition,
IEquatable,
interface,
Otherwise,
illustration,
StringBuilder,
instances,
operator,
references,
implementation,
scenarios,
Intern,
storage,
Compare,
Boolean,
Defines,
performance,
collection,
Some,
BinarySearch,
List,
Contains,
IndexOf,
LastIndexOf,
Remove,
Dictionary,
TKey,
TValue,
ContainsKey,
LinkedList,
argument,
integers,
Note,
IComparable,
CompareTo,
Temperature,
SortedList,
temperatures,
sequence,
whether,
variables,
constructor,
instantiate,
three,
objA,
objB,
runtime